什麼是 Stack 以及如何在沒有 Collection 的情況下在 Java 中實現 Stack?
已發表: 2022-06-27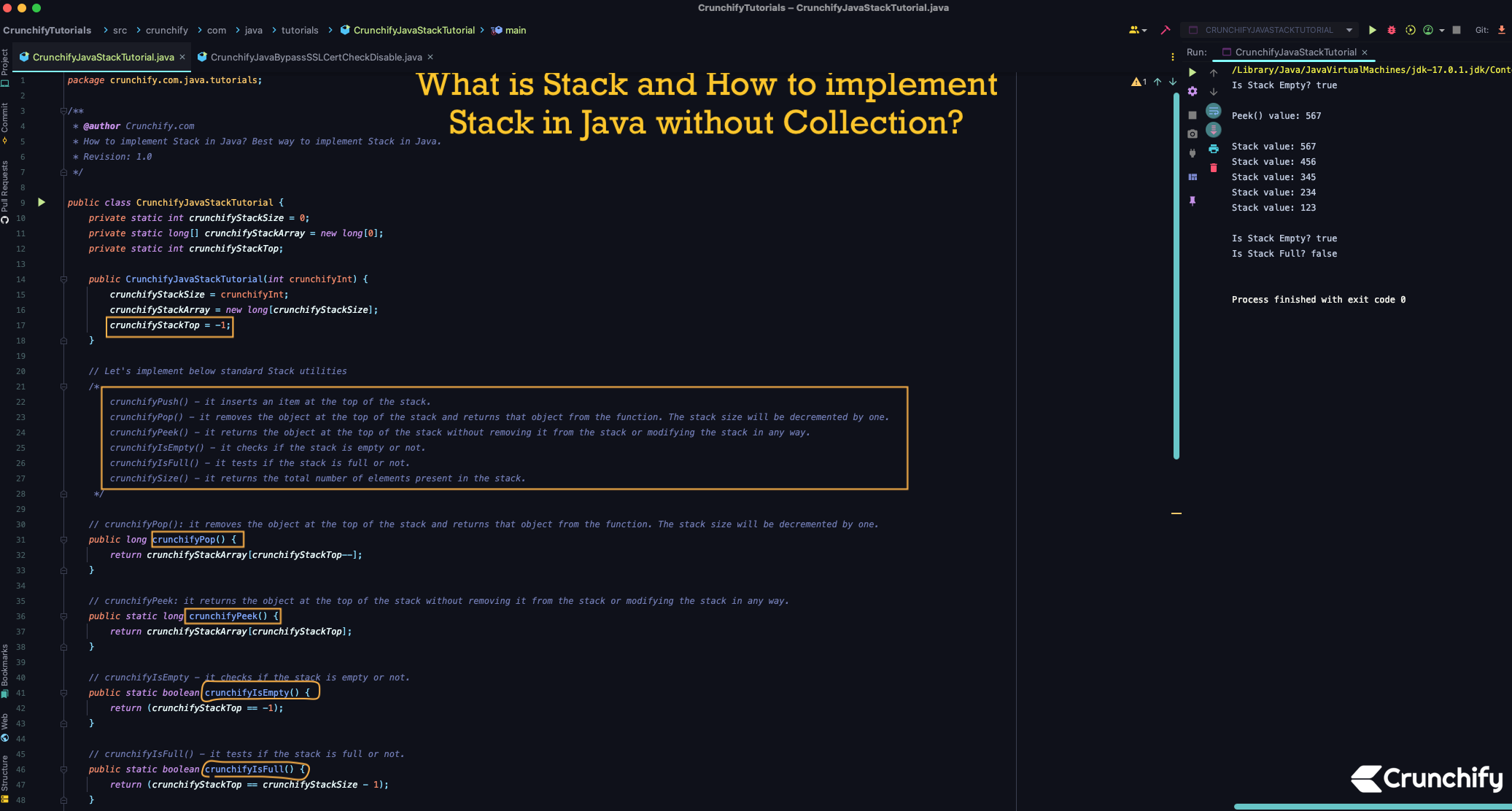
Java中的堆棧是什麼?
你聽說過LIFO嗎? 後進先出的概念? 好吧,Stack 是線性數據結構的 LIFO 實現。 這意味著,對像只能從一端插入或移除,或者換句話說只能從頂部插入或移除。
這是我們自己在 Java 中的 Stack 實現
我們將為 Java Stack 創建以下函數。 請注意:我們沒有使用任何內置的 Java Collection 類來實現 Stack。
我們將在下一個教程中使用 Java Collection for Stack。 現在已經出文章鏈接了。
- crunchifyPush() - 它在堆棧頂部插入一個項目。
- crunchifyPop() - 它刪除堆棧頂部的對象並從函數中返回該對象。 堆棧大小將減一。
- crunchifyPeek() - 它返回堆棧頂部的對象,而不將其從堆棧中刪除或以任何方式修改堆棧。
- crunchifyIsEmpty() - 檢查堆棧是否為空。
- crunchifyIsFull() - 它測試堆棧是否已滿。
- crunchifySize() - 它返回堆棧中存在的元素總數。
讓我們開始吧:
- 創建類 CrunchifyJavaStackTutorial.java
- 將以下代碼複製到您的 Eclipse 或 IntelliJ IDEA 中。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
package crunchify . com . java . tutorials ; /** * @author Crunchify.com * How to implement Stack in Java? Best way to implement Stack in Java. * Revision: 1.0 */ public class CrunchifyJavaStackTutorial { private static int crunchifyStackSize = 0 ; private static long [ ] crunchifyStackArray = new long [ 0 ] ; private static int crunchifyStackTop ; public CrunchifyJavaStackTutorial ( int crunchifyInt ) { crunchifyStackSize = crunchifyInt ; crunchifyStackArray = new long [ crunchifyStackSize ] ; crunchifyStackTop = - 1 ; } // Let's implement below standard Stack utilities /* crunchifyPush() - it inserts an item at the top of the stack. crunchifyPop() - it removes the object at the top of the stack and returns that object from the function. The stack size will be decremented by one. crunchifyPeek() - it returns the object at the top of the stack without removing it from the stack or modifying the stack in any way. crunchifyIsEmpty() - it checks if the stack is empty or not. crunchifyIsFull() - it tests if the stack is full or not. crunchifySize() - it returns the total number of elements present in the stack. */ // crunchifyPop(): it removes the object at the top of the stack and returns that object from the function. The stack size will be decremented by one. public long crunchifyPop ( ) { return crunchifyStackArray [ crunchifyStackTop -- ] ; } // crunchifyPeek: it returns the object at the top of the stack without removing it from the stack or modifying the stack in any way. public static long crunchifyPeek ( ) { return crunchifyStackArray [ crunchifyStackTop ] ; } // crunchifyIsEmpty - it checks if the stack is empty or not. public static boolean crunchifyIsEmpty ( ) { return ( crunchifyStackTop == - 1 ) ; } // crunchifyIsFull() - it tests if the stack is full or not. public static boolean crunchifyIsFull ( ) { return ( crunchifyStackTop == crunchifyStackSize - 1 ) ; } // crunchifyPush() - it inserts an item at the top of the stack. public void crunchifyPush ( long j ) { crunchifyStackArray [ ++ crunchifyStackTop ] = j ; } // crunchifySize() - it returns the total number of elements present in the stack. public static int crunchifySize ( ) { return crunchifyStackTop + 1 ; } public static void main ( String [ ] crunchifyArgs ) { CrunchifyJavaStackTutorial crunchifyStack = new CrunchifyJavaStackTutorial ( 5 ) ; crunchifyPrint ( "Is Stack Empty? " + crunchifyIsEmpty ( ) + "\n" ) ; crunchifyStack . crunchifyPush ( 123 ) ; crunchifyStack . crunchifyPush ( 234 ) ; crunchifyStack . crunchifyPush ( 345 ) ; crunchifyStack . crunchifyPush ( 456 ) ; crunchifyStack . crunchifyPush ( 567 ) ; crunchifyPrint ( "Peek() value: " + crunchifyPeek ( ) + "\n" ) ; while ( ! crunchifyIsEmpty ( ) ) { long crunchifyValue = crunchifyStack . crunchifyPop ( ) ; crunchifyPrint ( "Stack value: " + crunchifyValue ) ; } crunchifyPrint ( "" ) ; crunchifyPrint ( "Is Stack Empty? " + crunchifyIsEmpty ( ) ) ; crunchifyPrint ( "Is Stack Full? " + crunchifyIsFull ( ) + "\n" ) ; } // Simple Crunchify Print Utility private static void crunchifyPrint ( Object crunchifyValue ) { System . out . println ( crunchifyValue ) ; } } |
運行 Java 程序:
只需將上面的程序作為 Java 應用程序運行,您應該會看到如下結果。

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Is Stack Empty ? true Peek ( ) value : 567 Stack value : 567 Stack value : 456 Stack value : 345 Stack value : 234 Stack value : 123 Is Stack Empty ? true Is Stack Full ? false Process finished with exit code 0 |
如果您有任何問題或在 Java 程序上運行時遇到任何異常,請告訴我,我非常樂意與您一起調試。