Vue.js Web 开发中的有用工具
已发表: 2022-03-10在处理新项目时,根据应用程序的使用方式,有一些必要的功能。 例如,如果您要存储特定于用户的数据,则需要处理身份验证,这将需要设置需要验证的表单。 身份验证和表单验证等事情很常见; 有可能适合您的用例的解决方案。
为了正确利用您的开发时间,最好使用可用的东西,而不是发明您的。
作为一名新开发人员,您可能不了解 Vue 生态系统为您提供的所有内容。 本文将对此有所帮助; 它将涵盖某些有用的工具,这些工具将帮助您构建更好的 Vue 应用程序。
注意:这些库有替代品,本文绝不会将这几个放在其他库之上。 他们只是我合作过的人。
本教程面向刚开始学习 Vue 或已经具备 Vue 基础知识的初学者。 本教程中使用的所有代码片段都可以在我的 GitHub 上找到。
Vue通知
在用户交互过程中,通常需要向用户显示成功消息、错误消息或随机信息。 在本节中,我们将了解如何使用vue-notification
向用户显示消息和警告。 这个包提供了一个带有漂亮动画/过渡的界面,用于在您的应用程序中向您的用户显示错误、一般信息和成功消息,并且不需要大量配置即可启动和运行。
安装
您可以根据项目的包管理器使用 Yarn 或 NPM 在项目中安装vue-notification
纱
yarn add vue-notification
npm
npm install --save vue-notification
安装完成后,接下来就是将其添加到应用程序的入口点main.js文件中。
main.js
//several lines of existing code in the file import Notifications from 'vue-notification' Vue.use(Notifications)
此时,我们只需要在App.vue文件中添加通知组件,就可以在我们的应用中显示通知。 我们将这个组件添加到App.vue文件的原因是为了避免在我们的应用程序中重复,因为无论用户在我们的应用程序中的页面是什么, App.vue中的组件(例如页眉和页脚组件)总是能得到的。 这需要在我们需要向用户显示通知的每个文件中注册通知组件。
应用程序.vue
<template> <div> <div> <router-link to="/">Home</router-link> | <router-link to="/about">Notifications</router-link> </div> <notifications group="demo"/> <router-view /> </div> </template>
在这里,我们添加了这个组件的一个实例,它接受一个group
属性,该属性将用于对我们拥有的不同类型的通知进行分组。 这是因为通知组件接受许多指示组件行为方式的道具,我们将研究其中的一些。
-
group
此道具用于指定您的应用中可能拥有的不同类型的通知。 例如,您可能决定使用不同的样式和行为,具体取决于通知应该服务的目的、表单验证、API 响应等。 -
type
这个道具接受一个值,作为我们应用程序中每种通知类型的“类名”,示例可以包括success
、error
和warn
。 如果我们使用其中任何一种作为通知类型,我们可以通过使用此类格式vue-notification + '.' + type
轻松设置组件的样式。vue-notification + '.' + type
,即.vue-notification.warn
forwarn
等等。 -
duration
这个道具指定notification
组件在消失之前应该出现多长时间。 它接受一个数字作为以ms
为单位的值,如果您希望它保留在用户的屏幕上直到他们点击它,它也接受一个负数 (-1)。 -
position
此道具用于设置您希望通知出现在应用程序中的位置。 一些可用的选项是top left
、top right
、中top center
、bottom right
、bottom left
和bottom center
。
我们可以在App.vue中将这些道具添加到我们的组件中,所以它现在看起来像这样;
<template> <div> <div> <router-link to="/">Home</router-link> | <router-link to="/about">Notifications</router-link> </div> <notifications :group="group" :type="type" :duration="duration" :position="position" /> <router-view /> </div> </template> <script> export default { data() { return { duration: -1, group: "demo", position: "top center", type: "info", }; }, }; </script> <style> .vue-notification.info { border-left: 0; background-color: orange; } .vue-notification.success { border-left: 0; background-color: limegreen; } .vue-notification.error { border-left: 0; background-color: red; } </style>
我们还为我们将在应用程序中使用的不同通知类型添加样式。 请注意,除了group
之外,我们可以在想要显示通知时即时传递每个剩余的道具,并且它仍然会相应地工作。 要在任何 Vue 文件中显示通知,您可以执行以下操作。
vueFile.vue
this.$notify({ group: "demo", type: "error", text: "This is an error notification", });
在这里,我们在demo
的group
通知下创建type
error
的通知。 属性text
接受您希望通知包含的消息,在这种情况下,消息是“这是一个错误通知”。 这就是通知在您的应用程序中的样子。
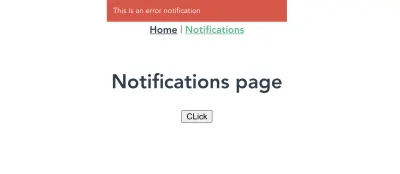
vue-notification
in action:在浏览器中显示错误通知。 (大预览)您可以在官方文档页面上找到其他可用的道具和其他配置通知的方法。
维利达
Web 上最常用的元素之一是表单元素( input[type='text']
、 input[type='email']
、 input[type='password']
等),并且总是有一个需要验证用户输入以确保他们发送正确的数据和/或在输入字段中使用正确的格式。 使用 Vuelidate,您可以向 Vue.js 应用程序中的表单添加验证,从而节省时间并从放入此包中的时间中受益。 我听说 Vuelidate 已经有一段时间了,但我有点不愿意看它,因为我认为它太复杂了,这意味着我要从头开始为我工作的应用程序中的大多数表单字段编写验证。
当我最终查看文档时,我发现入门并不难,我可以立即验证我的表单字段并继续下一步。
安装
您可以使用以下任何包管理器安装 Vuelidate。
纱
yarn add vuelidate
npm
npm install vuelidate --save
安装后,接下来就是将它添加到main.js文件中的应用程序配置中,以便您可以在 vue 文件中使用它。
import Vuelidate from 'vuelidate' Vue.use(Vuelidate)
假设您的应用中有一个看起来像这样的表单;
vuelidate.vue
<template> <form @submit.prevent="login" class="form"> <div class="input__container"> <label for="fullName" class="input__label">Full Name</label> <input type="text" name="fullName" v-model="form.fullName" class="input__field" /> </div> <div class="input__container"> <label for="email" class="input__label">Email</label> <input type="email" name="email" v-model="form.email" class="input__field" /> </div> <div class="input__container"> <label for="email" class="input__label">Age</label> <input type="number" name="age" v-model="form.age" class="input__field" /> </div> <div class="input__container"> <label for="password" class="input__label">Password</label> <input type="password" name="password" v-model="form.password" class="input__field" /> </div> <input type="submit" value="LOGIN" class="input__button" /> <p class="confirmation__text" v-if="submitted">Form clicked</p> </form> </template> <script> export default { data() { return { submitted: false, form: { email: null, fullName: null, age: null, password: null, }, }; }, methods: { login() { this.submitted = true; }, }, }; </script>
现在要验证这种类型的表单,您首先需要确定每个表单字段需要哪种类型的验证。 例如,您可以决定需要fullName
的最小长度为10
,最小年龄为18
。
Vuelidate 带有内置的验证器,我们只需要导入它即可使用。 我们还可以选择基于特定格式验证密码字段,例如Password should contain at least a lower case letter, an upper case letter, and a special character
。 我们可以编写自己的小验证器来执行此操作,并将其插入 Vuelidate 的插件列表中。
让我们一步一步来。
使用内置验证器
<script> import { required, minLength, minValue, email, } from "vuelidate/lib/validators"; export default { validations: { form: { email: { email, required, }, fullName: { minLength: minLength(10), required, }, age: { required, minValue: minValue(18), }, }, }, }; </script>
在这里,我们导入了一些我们需要正确验证表单的验证器。 我们还添加了一个validations
属性,我们在其中为要验证的每个表单字段定义验证规则。
此时,如果您检查应用程序的 devTools,您应该会看到如下所示的内容;
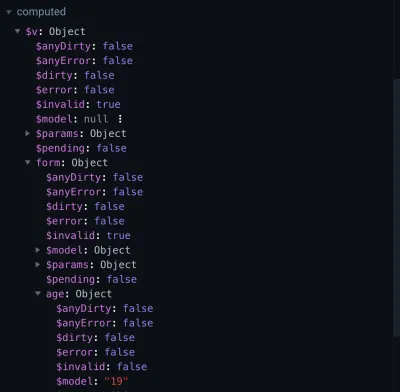
vuelidate
计算属性(大预览) $v
计算属性包含许多用于确认表单有效性的方法,但我们只关注其中的几个:
-
$invalid
检查表单是否通过所有验证。 -
email
检查该值是否为有效的电子邮件地址。 -
minValue
检查age
的值是否通过了minValue
检查。 -
minLength
验证fullName
的长度。 -
required
确保提供所有必填字段。
如果您输入的age
值小于验证中设置的最小年龄并检查$v.form.age.minValue
,它将设置为false
,这意味着输入字段中的值未通过minValue
验证检查.
使用自定义验证器
我们还需要验证我们的密码字段并确保它包含所需的格式,但 Vuelidate 没有我们可以用来实现此目的的内置验证器。 我们可以编写自己的自定义验证器,使用 RegEx 执行此操作。 这个自定义验证器看起来像这样;
<script> import { required, minLength, minValue, email, } from "vuelidate/lib/validators"; export default { validations: { form: { //existing validator rules password: { required, validPassword(password) { let regExp = /^(?=.*[0-9])(?=.*[!@#$%^&*])(?=.*[AZ]+)[a-zA-Z0-9!@#$%^&*]{6,}$/; return regExp.test(password); }, }, }, }, }; </script>
在这里,我们创建了一个自定义验证器,它使用正则表达式来检查密码是否包含以下内容;

- 至少有一个大写字母;
- 至少一个小写字母;
- 至少一个特殊字符;
- 至少一个数字;
- 最小长度必须为 6。
如果您尝试输入任何不符合上述任何要求的密码,则validPassword
将设置为false
。
现在我们确定我们的验证工作正常,我们必须显示适当的错误消息,以便用户知道他们为什么不能继续。 这看起来像这样:
<template> <form @submit.prevent="login" class="form"> <div class="input__container"> <label for="fullName" class="input__label">Full Name</label> <input type="text" name="fullName" v-model="form.fullName" class="input__field" /> <p class="error__text" v-if="!$v.form.fullName.required"> This field is required </p> </div> <div class="input__container"> <label for="email" class="input__label">Email</label> <input type="email" name="email" v-model="form.email" class="input__field" /> <p class="error__text" v-if="!$v.form.email.required"> This field is required </p> <p class="error__text" v-if="!$v.form.email.email"> This email is invalid </p> </div> <div class="input__container"> <label for="email" class="input__label">Age</label> <input type="number" name="age" v-model="form.age" class="input__field" /> <p class="error__text" v-if="!$v.form.age.required"> This field is required </p> </div> <div class="input__container"> <label for="password" class="input__label">Password</label> <input type="password" name="password" v-model="form.password" class="input__field" /> <p class="error__text" v-if="!$v.form.password.required"> This field is required </p> <p class="error__text" v-else-if="!$v.form.password.validPassword"> Password should contain at least a lower case letter, an upper case letter, a number and a special character </p> </div> <input type="submit" value="LOGIN" class="input__button" /> </form> </template>
在这里,我们添加一个段落,该段落显示一个文本,告诉用户一个字段是必需的,电子邮件的输入值无效或密码不包含所需的字符。 如果我们在您的浏览器中查看此内容,您会看到错误已经出现在每个输入字段下。
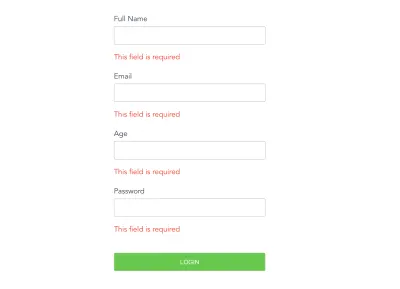
这对用户体验不利,因为用户尚未与表单交互,因此至少在用户尝试提交表单之前,错误文本不应该是可见的。 为了解决这个问题,我们将submitted
添加到显示错误文本所需的条件,并在用户单击提交按钮时将submitted
的值切换为true
。
<template> <form @submit.prevent="login" class="form"> <div class="input__container"> <label for="fullName" class="input__label">Full Name</label> <input type="text" name="fullName" v-model="form.fullName" class="input__field" /> <p class="error__text" v-if="submitted && !$v.form.fullName.required"> This field is required </p> </div> <div class="input__container"> <label for="email" class="input__label">Email</label> <input type="email" name="email" v-model="form.email" class="input__field" /> <p class="error__text" v-if="submitted && !$v.form.email.required"> This field is required </p> <p class="error__text" v-if="submitted && !$v.form.email.email"> This email is invalid </p> </div> <div class="input__container"> <label for="email" class="input__label">Age</label> <input type="number" name="age" v-model="form.age" class="input__field" /> <p class="error__text" v-if="submitted && !$v.form.age.required"> This field is required </p> </div> <div class="input__container"> <label for="password" class="input__label">Password</label> <input type="password" name="password" v-model="form.password" class="input__field" /> <p class="error__text" v-if="submitted && !$v.form.password.required"> This field is required </p> <p class="error__text" v-else-if="submitted && !$v.form.password.validPassword" > Password should contain at least a lower case letter, an upper case letter, a number and a special character </p> </div> <input type="submit" value="LOGIN" class="input__button" /> </form> </template>
现在错误文本在用户点击提交按钮之前不会出现,这对用户来说要好得多。 如果表单中输入的值不满足验证,则会出现每个验证错误。
最后,我们只想在表单上的所有验证都通过后处理用户的输入,我们可以做到这一点的一种方法是使用$v
计算属性中存在的form
上的$invalid
属性。 让我们来看看如何做到这一点:
methods: { login() { this.submitted = true; let invalidForm = this.$v.form.$invalid; //check that every field in this form has been entered correctly. if (!invalidForm) { // process the form data } }, },
在这里,我们正在检查以确保表格已完全填写并正确填写。 如果返回false
,则表示表单有效,我们可以处理表单中的数据,但如果返回true
,则表示表单仍然无效,用户仍然需要处理表单中的一些错误。 我们还可以根据您的偏好使用此属性来禁用或设置提交按钮的样式。
Vuex-持久状态
在开发过程中,有些情况下您会在 Vuex 商店中存储用户信息和令牌等数据。 但是,如果您的用户尝试从浏览器刷新您的应用程序或从浏览器的 URL 选项卡输入新路由并且您的应用程序的当前状态随之丢失,您的 Vuex 存储数据将不会保留。 如果路由受到导航保护的保护,这会导致用户被重定向到登录页面,这对于您的应用来说是异常行为。 这可以通过vuex-persistedstate
修复,让我们看看如何。
安装
您可以使用以下两种方法中的任何一种来安装此插件:
纱
yarn add vuex-persistedstate
npm
npm install --save vuex-persistedstate
安装过程完成后,下一步是配置此插件,以便在您的 Vuex 商店中使用。
import Vue from 'vue' import Vuex from 'vuex' import createPersistedState from "vuex-persistedstate"; Vue.use(Vuex) export default new Vuex.Store({ state: {}, mutations: {}, actions: {}, modules: {}, plugins: [createPersistedState()] })
此时,我们所有的 Vuex Store 都将存储在 localStorage 中(默认情况下),但vuex-persistedstate
带有使用sessionStorage
或cookies
的选项。
import Vue from 'vue' import Vuex from 'vuex' import createPersistedState from "vuex-persistedstate"; Vue.use(Vuex) export default new Vuex.Store({ state: {}, mutations: {}, actions: {}, modules: {}, // changes storage to sessionStorage plugins: [createPersistedState({ storage: window.sessionStorage }); ] })
为了确认我们的商店在刷新或关闭浏览器选项卡后仍然存在,让我们将我们的商店更新为如下所示:
import Vue from 'vue' import Vuex from 'vuex' import createPersistedState from "vuex-persistedstate"; Vue.use(Vuex) export default new Vuex.Store({ state: { user: null }, mutations: { SET_USER(state, user) { state.user = user } }, actions: { getUser({ commit }, userInfo) { commit('SET_USER', userInfo) } }, plugins: [createPersistedState()] })
在这里,我们添加了一个user
状态,它将存储上一节中创建的表单中的用户数据。 我们还添加了一个SET_USER
突变,用于修改user
状态。 最后,我们添加一个getUser
操作,该操作将接收用户对象并将其传递给SET_USER
突变属性。 接下来是在成功验证我们的表单后发送此操作。 这看起来像这样:
methods: { login() { this.submitted = true; let invalidForm = this.$v.form.$invalid; let form = this.form; //check that every field in this form has been entered correctly. if (!invalidForm) { // process the form data this.$store.dispatch("getUser", form); } }, },
现在,如果您正确填写表单、提交并打开浏览器 devTools 的应用程序选项卡中的localStorage
部分,您应该会看到如下所示的vuex
属性:
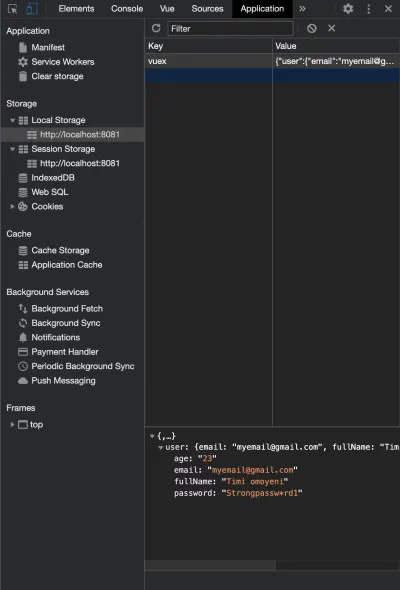
此时,如果您刷新浏览器或在新选项卡中打开您的应用程序,您的user
状态仍将在这些选项卡/会话(在 localStorage 上)中持续存在。
结论
有很多库在 Vuejs Web 开发中非常有用,有时很难选择要使用的库或在哪里找到它们。 以下链接包含您可以在 Vue.js 应用程序中使用的库。
- vuejsexamples.com。
- madewithvuejs.com。
在搜索“库”时,通常有不止一个库会执行您在应用程序中尝试实现的相同操作,重要的是确保您选择的选项适合您并由它的创建者,所以它不会导致您的应用程序中断。
更多资源
- “Vue.js 通知”,官方文档,GitHub
- “Vuelidate”,官方网站
- “使用 Vuelidate 在一小时内完成表单验证”,CSS-Tricks 的 Sarah Drasner
- “
vuex-persistedstate
”,纱线