Yığın nedir ve Java'da Koleksiyonsuz Yığın nasıl uygulanır?
Yayınlanan: 2022-06-27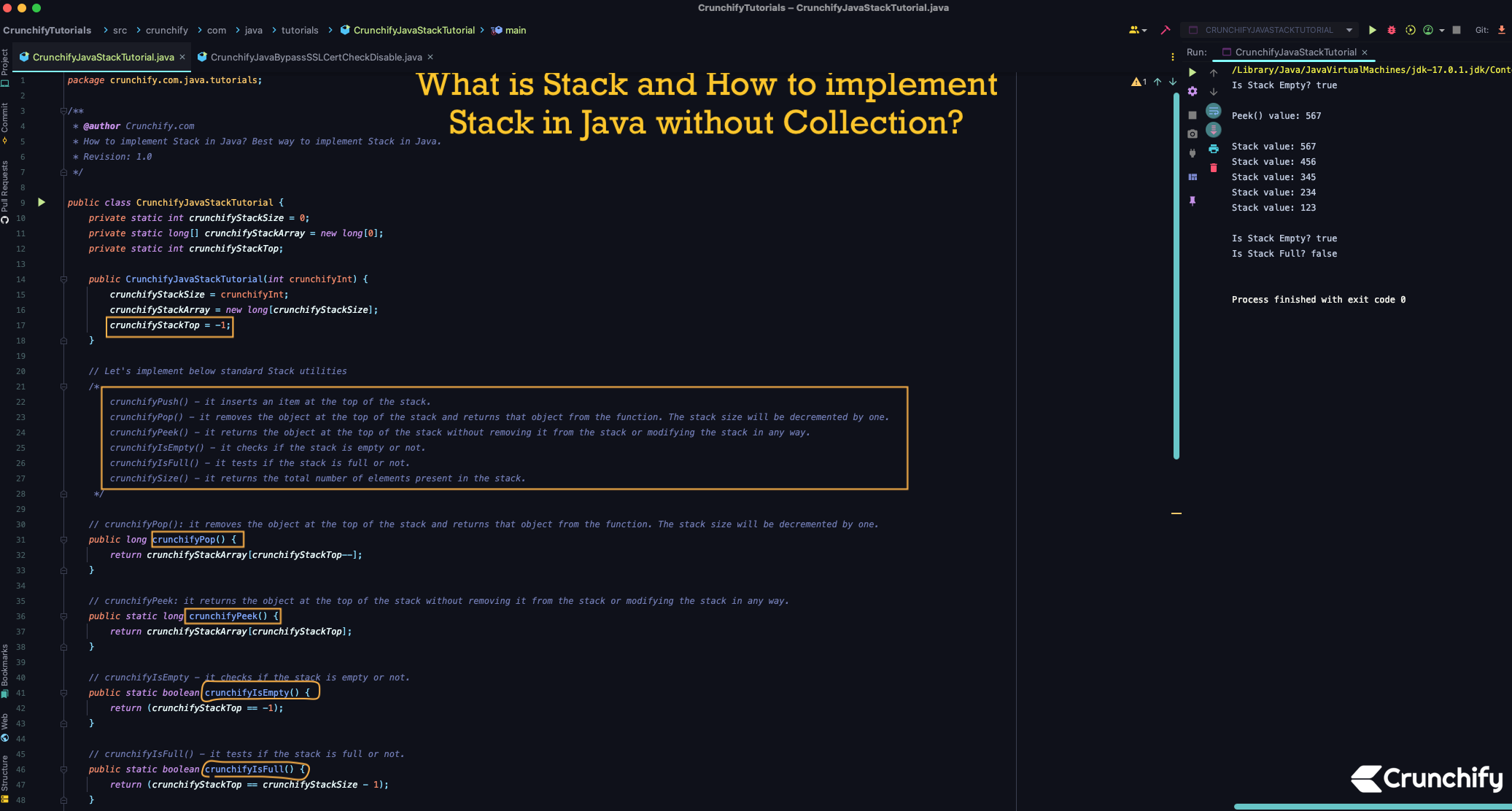
Java'da Yığın nedir?
LIFO'yu duydunuz mu? Son Giren İlk Çıkar konsepti? Eh, Stack, doğrusal Veri Yapısının bir LIFO uygulamasıdır. Bu, Nesnelerin yalnızca bir uçtan VEYA başka bir deyişle yalnızca üstten eklenebileceği veya kaldırılabileceği anlamına gelir.
İşte Java'da kendi Stack uygulamamız
Java Stack için aşağıdaki fonksiyonları oluşturacağız. Lütfen buraya dikkat edin: Yığın uygulaması için herhangi bir yerleşik Java Koleksiyonu sınıfı kullanmıyoruz.
Bir sonraki eğitimde Stack için Java Koleksiyonunu kullanacağız. Şimdi çıktı Makale bağlantısı.
- crunchifyPush() – yığının en üstüne bir öğe ekler.
- crunchifyPop() - yığının en üstündeki nesneyi kaldırır ve o nesneyi işlevden döndürür. Yığın boyutu bir azaltılacaktır.
- crunchifyPeek() – yığının üstündeki nesneyi yığından çıkarmadan veya yığını herhangi bir şekilde değiştirmeden döndürür.
- crunchifyIsEmpty() – yığının boş olup olmadığını kontrol eder.
- crunchifyIsFull() - yığının dolu olup olmadığını test eder.
- crunchifySize() - yığında bulunan toplam öğe sayısını döndürür.
Başlayalım:
- CrunchifyJavaStackTutorial.java sınıfı oluşturun
- Aşağıdaki kodu Eclipse veya IntelliJ IDEA'nıza kopyalayın.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
package crunchify . com . java . tutorials ; /** * @author Crunchify.com * How to implement Stack in Java? Best way to implement Stack in Java. * Revision: 1.0 */ public class CrunchifyJavaStackTutorial { private static int crunchifyStackSize = 0 ; private static long [ ] crunchifyStackArray = new long [ 0 ] ; private static int crunchifyStackTop ; public CrunchifyJavaStackTutorial ( int crunchifyInt ) { crunchifyStackSize = crunchifyInt ; crunchifyStackArray = new long [ crunchifyStackSize ] ; crunchifyStackTop = - 1 ; } // Let's implement below standard Stack utilities /* crunchifyPush() - it inserts an item at the top of the stack. crunchifyPop() - it removes the object at the top of the stack and returns that object from the function. The stack size will be decremented by one. crunchifyPeek() - it returns the object at the top of the stack without removing it from the stack or modifying the stack in any way. crunchifyIsEmpty() - it checks if the stack is empty or not. crunchifyIsFull() - it tests if the stack is full or not. crunchifySize() - it returns the total number of elements present in the stack. */ // crunchifyPop(): it removes the object at the top of the stack and returns that object from the function. The stack size will be decremented by one. public long crunchifyPop ( ) { return crunchifyStackArray [ crunchifyStackTop -- ] ; } // crunchifyPeek: it returns the object at the top of the stack without removing it from the stack or modifying the stack in any way. public static long crunchifyPeek ( ) { return crunchifyStackArray [ crunchifyStackTop ] ; } // crunchifyIsEmpty - it checks if the stack is empty or not. public static boolean crunchifyIsEmpty ( ) { return ( crunchifyStackTop == - 1 ) ; } // crunchifyIsFull() - it tests if the stack is full or not. public static boolean crunchifyIsFull ( ) { return ( crunchifyStackTop == crunchifyStackSize - 1 ) ; } // crunchifyPush() - it inserts an item at the top of the stack. public void crunchifyPush ( long j ) { crunchifyStackArray [ ++ crunchifyStackTop ] = j ; } // crunchifySize() - it returns the total number of elements present in the stack. public static int crunchifySize ( ) { return crunchifyStackTop + 1 ; } public static void main ( String [ ] crunchifyArgs ) { CrunchifyJavaStackTutorial crunchifyStack = new CrunchifyJavaStackTutorial ( 5 ) ; crunchifyPrint ( "Is Stack Empty? " + crunchifyIsEmpty ( ) + "\n" ) ; crunchifyStack . crunchifyPush ( 123 ) ; crunchifyStack . crunchifyPush ( 234 ) ; crunchifyStack . crunchifyPush ( 345 ) ; crunchifyStack . crunchifyPush ( 456 ) ; crunchifyStack . crunchifyPush ( 567 ) ; crunchifyPrint ( "Peek() value: " + crunchifyPeek ( ) + "\n" ) ; while ( ! crunchifyIsEmpty ( ) ) { long crunchifyValue = crunchifyStack . crunchifyPop ( ) ; crunchifyPrint ( "Stack value: " + crunchifyValue ) ; } crunchifyPrint ( "" ) ; crunchifyPrint ( "Is Stack Empty? " + crunchifyIsEmpty ( ) ) ; crunchifyPrint ( "Is Stack Full? " + crunchifyIsFull ( ) + "\n" ) ; } // Simple Crunchify Print Utility private static void crunchifyPrint ( Object crunchifyValue ) { System . out . println ( crunchifyValue ) ; } } |
Java Programını Çalıştırın:
Sadece yukarıdaki programı bir Java Uygulaması olarak çalıştırın ve sonucu aşağıdaki gibi görmelisiniz.

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Is Stack Empty ? true Peek ( ) value : 567 Stack value : 567 Stack value : 456 Stack value : 345 Stack value : 234 Stack value : 123 Is Stack Empty ? true Is Stack Full ? false Process finished with exit code 0 |
Herhangi bir sorunuz varsa veya Java programının üzerinde çalışan herhangi bir istisnayla karşılaşırsanız bana bildirin ve bunu sizinle hata ayıklamaktan mutluluk duyarım.