Javaで国のリストを見つけてアルファベット順に並べ替える方法は?
公開: 2022-03-06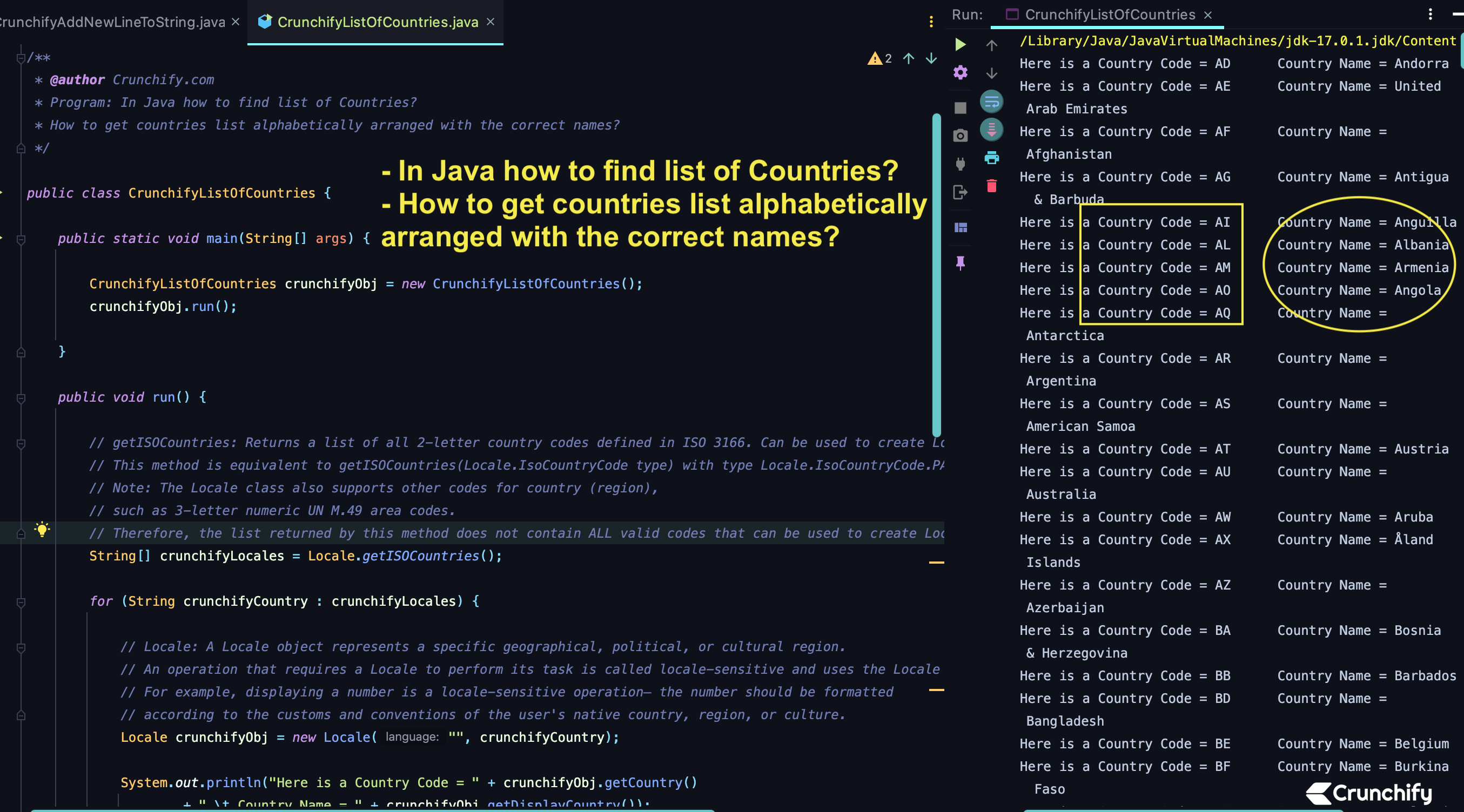
Javaで国のリストを見つける方法は? 以下の質問のいずれかがある場合は、適切な場所にいます。
- Javaで国のリストを取得する最良の方法は?
- 国のリストを正しい名前でアルファベット順に並べるにはどうすればよいですか?
始めましょう:
- JavaクラスCrunchifyListOfCountries.javaを作成します。
- 以下のコードをファイルに入れます。
ロジックは次のとおりです。
- CloneableおよびSerializableインターフェイスを実装するLocaleクラスを使用します。
- ロケールクラスはAPIのリストを提供し、getCountry()と
getDisplayCountry()
を繰り返し処理して、すべての国とコードのリストを取得します。 - 次のメソッドgetCountriesListInAlphabetical()では、ListメソッドとListIteratorメソッドを使用して反復し、
sort()
メソッドを使用してリストをアルファベット順に並べ替えます。
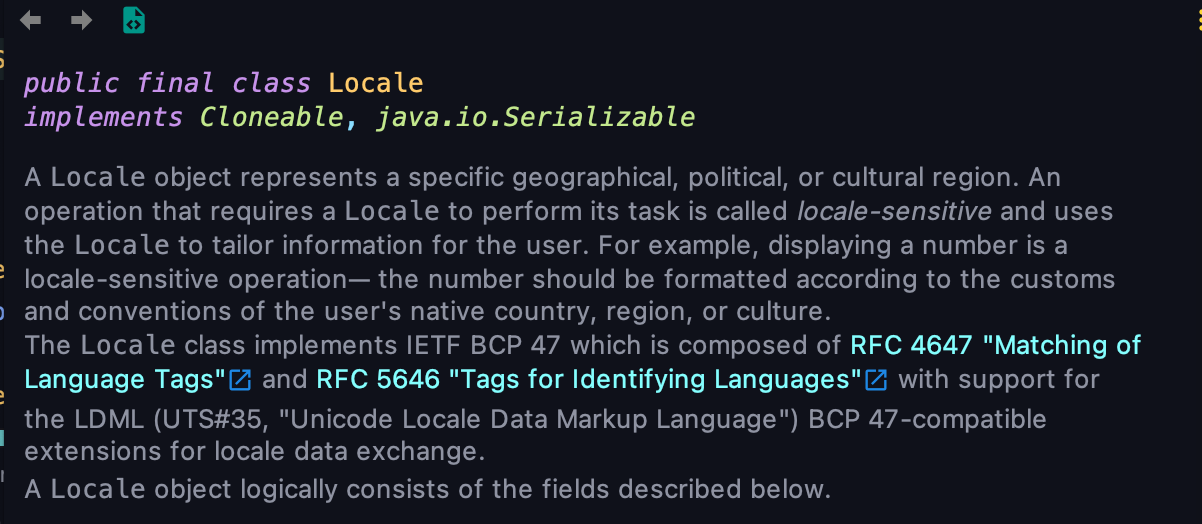
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 |
package crunchify . com . java . tutorials ; import java . util . * ; /** * @author Crunchify.com * Program: In Java how to find list of Countries? * How to get countries list alphabetically arranged with the correct names? */ public class CrunchifyListOfCountries { public static void main ( String [ ] args ) { CrunchifyListOfCountries crunchifyObj = new CrunchifyListOfCountries ( ) ; crunchifyObj . run ( ) ; } public void run ( ) { // getISOCountries: Returns a list of all 2-letter country codes defined in ISO 3166. Can be used to create Locales. // This method is equivalent to getISOCountries(Locale.IsoCountryCode type) with type Locale.IsoCountryCode.PART1_ALPHA2. // Note: The Locale class also supports other codes for country (region), // such as 3-letter numeric UN M.49 area codes. // Therefore, the list returned by this method does not contain ALL valid codes that can be used to create Locales. String [ ] crunchifyLocales = Locale . getISOCountries ( ) ; for ( String crunchifyCountry : crunchifyLocales ) { // Locale: A Locale object represents a specific geographical, political, or cultural region. // An operation that requires a Locale to perform its task is called locale-sensitive and uses the Locale to tailor information for the user. // For example, displaying a number is a locale-sensitive operation— the number should be formatted // according to the customs and conventions of the user's native country, region, or culture. Locale crunchifyObj = new Locale ( "" , crunchifyCountry ) ; System . out . println ( "Here is a Country Code = " + crunchifyObj . getCountry ( ) + " \t Country Name = " + crunchifyObj . getDisplayCountry ( ) ) ; } System . out . println ( "\n\nCountry List in Alphabetical Order:\n" ) ; getCountriesListInAlphabetical ( ) ; System . out . println ( "CrunchifyListOfCountries Finished..." ) ; } // Country List in Alphabetical Order public void getCountriesListInAlphabetical ( ) { List < String > crunchifyList = new ArrayList < String > ( ) ; String [ ] locales = Locale . getISOCountries ( ) ; for ( String countryCode : locales ) { Locale obj = new Locale ( "" , countryCode ) ; crunchifyList . add ( obj . getDisplayCountry ( ) ) ; } // sort(): Sorts the specified list into ascending order, // according to the natural ordering of its elements. // All elements in the list must implement the Comparable interface. Furthermore, // all elements in the list must be mutually comparable // (that is, e1.compareTo(e2) must not throw a ClassCastException for any elements e1 and e2 in the list). Collections . sort ( crunchifyList ) ; // listIterator(): Returns a list iterator over the elements in this list (in proper sequence). ListIterator < String > crunchifyListIterator = crunchifyList . listIterator ( ) ; while ( crunchifyListIterator . hasNext ( ) ) { // next(): Returns the next element in the list and advances the cursor position. This method may be called repeatedly to iterate through the list, or intermixed with calls to previous to go back and forth. // (Note that alternating calls to next and previous will return the same element repeatedly.) System . out . println ( crunchifyListIterator . next ( ) ) ; } } } |
上記のプログラムをJavaアプリケーションとして実行するだけで、以下のような結果が表示されます。
IntelliJ IDEAの結果:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 243 244 245 246 247 248 249 250 251 252 253 254 255 256 257 258 259 260 261 262 263 264 265 266 267 268 269 270 271 272 273 274 275 276 277 278 279 280 281 282 283 284 285 286 287 288 289 290 291 292 293 294 295 296 297 298 299 300 301 302 303 304 305 306 307 308 309 310 311 312 313 314 315 316 317 318 319 320 321 322 323 324 325 326 327 328 329 330 331 332 333 334 335 336 337 338 339 340 341 342 343 344 345 346 347 348 349 350 351 352 353 354 355 356 357 358 359 360 361 362 363 364 365 366 367 368 369 370 371 372 373 374 375 376 377 378 379 380 381 382 383 384 385 386 |
Here is a Country Code = AD Country Name = Andorra Here is a Country Code = AE Country Name = United Arab Emirates Here is a Country Code = AF Country Name = Afghanistan Here is a Country Code = AG Country Name = Antigua & Barbuda Here is a Country Code = AI Country Name = Anguilla Here is a Country Code = AL Country Name = Albania Here is a Country Code = AM Country Name = Armenia Here is a Country Code = AO Country Name = Angola Here is a Country Code = AQ Country Name = Antarctica Here is a Country Code = AR Country Name = Argentina Here is a Country Code = AS Country Name = American Samoa Here is a Country Code = AT Country Name = Austria Here is a Country Code = AU Country Name = Australia Here is a Country Code = AW Country Name = Aruba Here is a Country Code = AX Country Name = Å land Islands Here is a Country Code = AZ Country Name = Azerbaijan Here is a Country Code = BA Country Name = Bosnia & Herzegovina Here is a Country Code = BB Country Name = Barbados Here is a Country Code = BD Country Name = Bangladesh Here is a Country Code = BE Country Name = Belgium Here is a Country Code = BF Country Name = Burkina Faso Here is a Country Code = BG Country Name = Bulgaria Here is a Country Code = BH Country Name = Bahrain Here is a Country Code = BI Country Name = Burundi Here is a Country Code = BJ Country Name = Benin Here is a Country Code = BL Country Name = St . Barth e lemy Here is a Country Code = BM Country Name = Bermuda Here is a Country Code = BN Country Name = Brunei Here is a Country Code = BO Country Name = Bolivia Here is a Country Code = BQ Country Name = Caribbean Netherlands Here is a Country Code = BR Country Name = Brazil Here is a Country Code = BS Country Name = Bahamas Here is a Country Code = BT Country Name = Bhutan Here is a Country Code = BV Country Name = Bouvet Island Here is a Country Code = BW Country Name = Botswana Here is a Country Code = BY Country Name = Belarus Here is a Country Code = BZ Country Name = Belize Here is a Country Code = CA Country Name = Canada Here is a Country Code = CC Country Name = Cocos ( Keeling ) Islands Here is a Country Code = CD Country Name = Congo - Kinshasa Here is a Country Code = CF Country Name = Central African Republic Here is a Country Code = CG Country Name = Congo - Brazzaville Here is a Country Code = CH Country Name = Switzerland Here is a Country Code = CI Country Name = C o te d ’ Ivoire Here is a Country Code = CK Country Name = Cook Islands Here is a Country Code = CL Country Name = Chile Here is a Country Code = CM Country Name = Cameroon Here is a Country Code = CN Country Name = China Here is a Country Code = CO Country Name = Colombia Here is a Country Code = CR Country Name = Costa Rica Here is a Country Code = CU Country Name = Cuba Here is a Country Code = CV Country Name = Cape Verde Here is a Country Code = CW Country Name = Cura c ao Here is a Country Code = CX Country Name = Christmas Island Here is a Country Code = CY Country Name = Cyprus Here is a Country Code = CZ Country Name = Czechia Here is a Country Code = DE Country Name = Germany Here is a Country Code = DJ Country Name = Djibouti Here is a Country Code = DK Country Name = Denmark Here is a Country Code = DM Country Name = Dominica Here is a Country Code = DO Country Name = Dominican Republic Here is a Country Code = DZ Country Name = Algeria Here is a Country Code = EC Country Name = Ecuador Here is a Country Code = EE Country Name = Estonia Here is a Country Code = EG Country Name = Egypt Here is a Country Code = EH Country Name = Western Sahara Here is a Country Code = ER Country Name = Eritrea Here is a Country Code = ES Country Name = Spain Here is a Country Code = ET Country Name = Ethiopia Here is a Country Code = FI Country Name = Finland Here is a Country Code = FJ Country Name = Fiji Here is a Country Code = FK Country Name = Falkland Islands Here is a Country Code = FM Country Name = Micronesia Here is a Country Code = FO Country Name = Faroe Islands Here is a Country Code = FR Country Name = France Here is a Country Code = GA Country Name = Gabon Here is a Country Code = GB Country Name = United Kingdom Here is a Country Code = GD Country Name = Grenada Here is a Country Code = GE Country Name = Georgia Here is a Country Code = GF Country Name = French Guiana Here is a Country Code = GG Country Name = Guernsey Here is a Country Code = GH Country Name = Ghana Here is a Country Code = GI Country Name = Gibraltar Here is a Country Code = GL Country Name = Greenland Here is a Country Code = GM Country Name = Gambia Here is a Country Code = GN Country Name = Guinea Here is a Country Code = GP Country Name = Guadeloupe Here is a Country Code = GQ Country Name = Equatorial Guinea Here is a Country Code = GR Country Name = Greece Here is a Country Code = GS Country Name = South Georgia & South Sandwich Islands Here is a Country Code = GT Country Name = Guatemala Here is a Country Code = GU Country Name = Guam Here is a Country Code = GW Country Name = Guinea - Bissau Here is a Country Code = GY Country Name = Guyana Here is a Country Code = HK Country Name = Hong Kong SAR China Here is a Country Code = HM Country Name = Heard & McDonald Islands Here is a Country Code = HN Country Name = Honduras Here is a Country Code = HR Country Name = Croatia Here is a Country Code = HT Country Name = Haiti Here is a Country Code = HU Country Name = Hungary Here is a Country Code = ID Country Name = Indonesia Here is a Country Code = IE Country Name = Ireland Here is a Country Code = IL Country Name = Israel Here is a Country Code = IM Country Name = Isle of Man Here is a Country Code = IN Country Name = India Here is a Country Code = IO Country Name = British Indian Ocean Territory Here is a Country Code = IQ Country Name = Iraq Here is a Country Code = IR Country Name = Iran Here is a Country Code = IS Country Name = Iceland Here is a Country Code = IT Country Name = Italy Here is a Country Code = JE Country Name = Jersey Here is a Country Code = JM Country Name = Jamaica Here is a Country Code = JO Country Name = Jordan Here is a Country Code = JP Country Name = Japan Here is a Country Code = KE Country Name = Kenya Here is a Country Code = KG Country Name = Kyrgyzstan Here is a Country Code = KH Country Name = Cambodia Here is a Country Code = KI Country Name = Kiribati Here is a Country Code = KM Country Name = Comoros Here is a Country Code = KN Country Name = St . Kitts & Nevis Here is a Country Code = KP Country Name = North Korea Here is a Country Code = KR Country Name = South Korea Here is a Country Code = KW Country Name = Kuwait Here is a Country Code = KY Country Name = Cayman Islands Here is a Country Code = KZ Country Name = Kazakhstan Here is a Country Code = LA Country Name = Laos Here is a Country Code = LB Country Name = Lebanon Here is a Country Code = LC Country Name = St . Lucia Here is a Country Code = LI Country Name = Liechtenstein Here is a Country Code = LK Country Name = Sri Lanka Here is a Country Code = LR Country Name = Liberia Here is a Country Code = LS Country Name = Lesotho Here is a Country Code = LT Country Name = Lithuania Here is a Country Code = LU Country Name = Luxembourg Here is a Country Code = LV Country Name = Latvia Here is a Country Code = LY Country Name = Libya Here is a Country Code = MA Country Name = Morocco Here is a Country Code = MC Country Name = Monaco Here is a Country Code = MD Country Name = Moldova Here is a Country Code = ME Country Name = Montenegro Here is a Country Code = MF Country Name = St . Martin Here is a Country Code = MG Country Name = Madagascar Here is a Country Code = MH Country Name = Marshall Islands Here is a Country Code = MK Country Name = North Macedonia Here is a Country Code = ML Country Name = Mali Here is a Country Code = MM Country Name = Myanmar ( Burma ) Here is a Country Code = MN Country Name = Mongolia Here is a Country Code = MO Country Name = Macao SAR China Here is a Country Code = MP Country Name = Northern Mariana Islands Here is a Country Code = MQ Country Name = Martinique Here is a Country Code = MR Country Name = Mauritania Here is a Country Code = MS Country Name = Montserrat Here is a Country Code = MT Country Name = Malta Here is a Country Code = MU Country Name = Mauritius Here is a Country Code = MV Country Name = Maldives Here is a Country Code = MW Country Name = Malawi Here is a Country Code = MX Country Name = Mexico Here is a Country Code = MY Country Name = Malaysia Here is a Country Code = MZ Country Name = Mozambique Here is a Country Code = NA Country Name = Namibia Here is a Country Code = NC Country Name = New Caledonia Here is a Country Code = NE Country Name = Niger Here is a Country Code = NF Country Name = Norfolk Island Here is a Country Code = NG Country Name = Nigeria Here is a Country Code = NI Country Name = Nicaragua Here is a Country Code = NL Country Name = Netherlands Here is a Country Code = NO Country Name = Norway Here is a Country Code = NP Country Name = Nepal Here is a Country Code = NR Country Name = Nauru Here is a Country Code = NU Country Name = Niue Here is a Country Code = NZ Country Name = New Zealand Here is a Country Code = OM Country Name = Oman Here is a Country Code = PA Country Name = Panama Here is a Country Code = PE Country Name = Peru Here is a Country Code = PF Country Name = French Polynesia Here is a Country Code = PG Country Name = Papua New Guinea Here is a Country Code = PH Country Name = Philippines Here is a Country Code = PK Country Name = Pakistan Here is a Country Code = PL Country Name = Poland Here is a Country Code = PM Country Name = St . Pierre & Miquelon Here is a Country Code = PN Country Name = Pitcairn Islands Here is a Country Code = PR Country Name = Puerto Rico Here is a Country Code = PS Country Name = Palestinian Territories Here is a Country Code = PT Country Name = Portugal Here is a Country Code = PW Country Name = Palau Here is a Country Code = PY Country Name = Paraguay Here is a Country Code = QA Country Name = Qatar Here is a Country Code = RE Country Name = R e union Here is a Country Code = RO Country Name = Romania Here is a Country Code = RS Country Name = Serbia Here is a Country Code = RU Country Name = Russia Here is a Country Code = RW Country Name = Rwanda Here is a Country Code = SA Country Name = Saudi Arabia Here is a Country Code = SB Country Name = Solomon Islands Here is a Country Code = SC Country Name = Seychelles Here is a Country Code = SD Country Name = Sudan Here is a Country Code = SE Country Name = Sweden Here is a Country Code = SG Country Name = Singapore Here is a Country Code = SH Country Name = St . Helena Here is a Country Code = SI Country Name = Slovenia Here is a Country Code = SJ Country Name = Svalbard & Jan Mayen Here is a Country Code = SK Country Name = Slovakia Here is a Country Code = SL Country Name = Sierra Leone Here is a Country Code = SM Country Name = San Marino Here is a Country Code = SN Country Name = Senegal Here is a Country Code = SO Country Name = Somalia Here is a Country Code = SR Country Name = Suriname Here is a Country Code = SS Country Name = South Sudan Here is a Country Code = ST Country Name = S a o Tom e & Pr i ncipe Here is a Country Code = SV Country Name = El Salvador Here is a Country Code = SX Country Name = Sint Maarten Here is a Country Code = SY Country Name = Syria Here is a Country Code = SZ Country Name = Eswatini Here is a Country Code = TC Country Name = Turks & Caicos Islands Here is a Country Code = TD Country Name = Chad Here is a Country Code = TF Country Name = French Southern Territories Here is a Country Code = TG Country Name = Togo Here is a Country Code = TH Country Name = Thailand Here is a Country Code = TJ Country Name = Tajikistan Here is a Country Code = TK Country Name = Tokelau Here is a Country Code = TL Country Name = Timor - Leste Here is a Country Code = TM Country Name = Turkmenistan Here is a Country Code = TN Country Name = Tunisia Here is a Country Code = TO Country Name = Tonga Here is a Country Code = TR Country Name = Turkey Here is a Country Code = TT Country Name = Trinidad & Tobago Here is a Country Code = TV Country Name = Tuvalu Here is a Country Code = TW Country Name = Taiwan Here is a Country Code = TZ Country Name = Tanzania Here is a Country Code = UA Country Name = Ukraine Here is a Country Code = UG Country Name = Uganda Here is a Country Code = UM Country Name = U . S . Outlying Islands Here is a Country Code = US Country Name = United States Here is a Country Code = UY Country Name = Uruguay Here is a Country Code = UZ Country Name = Uzbekistan Here is a Country Code = VA Country Name = Vatican City Here is a Country Code = VC Country Name = St . Vincent & Grenadines Here is a Country Code = VE Country Name = Venezuela Here is a Country Code = VG Country Name = British Virgin Islands Here is a Country Code = VI Country Name = U . S . Virgin Islands Here is a Country Code = VN Country Name = Vietnam Here is a Country Code = VU Country Name = Vanuatu Here is a Country Code = WF Country Name = Wallis & Futuna Here is a Country Code = WS Country Name = Samoa Here is a Country Code = YE Country Name = Yemen Here is a Country Code = YT Country Name = Mayotte Here is a Country Code = ZA Country Name = South Africa Here is a Country Code = ZM Country Name = Zambia Here is a Country Code = ZW Country Name = Zimbabwe Country List in Alphabetical Order : Afghanistan Albania Algeria American Samoa Andorra Angola Anguilla Antarctica Antigua & Barbuda Argentina Armenia Aruba Australia Austria Azerbaijan Bahamas Bahrain Bangladesh Barbados Belarus Belgium Belize Benin Bermuda Bhutan Bolivia Bosnia & Herzegovina Botswana Bouvet Island Brazil British Indian Ocean Territory British Virgin Islands Brunei Bulgaria Burkina Faso Burundi Cambodia Cameroon Canada Cape Verde Caribbean Netherlands Cayman Islands Central African Republic Chad Chile China Christmas Island Cocos ( Keeling ) Islands Colombia Comoros Congo - Brazzaville Congo - Kinshasa Cook Islands Costa Rica Croatia Cuba Cura c ao Cyprus Czechia C o te d ’ Ivoire Denmark Djibouti Dominica Dominican Republic Ecuador Egypt El Salvador Equatorial Guinea Eritrea Estonia Eswatini Ethiopia Falkland Islands Faroe Islands Fiji Finland France French Guiana French Polynesia French Southern Territories Gabon Gambia Georgia Germany Ghana Gibraltar Greece Greenland Grenada Guadeloupe Guam Guatemala Guernsey Guinea Guinea - Bissau Guyana Haiti Heard & McDonald Islands Honduras Hong Kong SAR China Hungary Iceland India Indonesia Iran Iraq Ireland Isle of Man Israel Italy Jamaica Japan Jersey Jordan Kazakhstan Kenya Kiribati Kuwait Kyrgyzstan Laos Latvia Lebanon Lesotho Liberia . . . . . . And so on . . . . CrunchifyListOfCountries Finished . . . Process finished with exit code 0 |
上記のプログラムの実行中に問題が発生した場合はお知らせください。

ハッピーコーディング。