Was ist Stack und wie implementiert man Stack in Java ohne Collection?
Veröffentlicht: 2022-06-27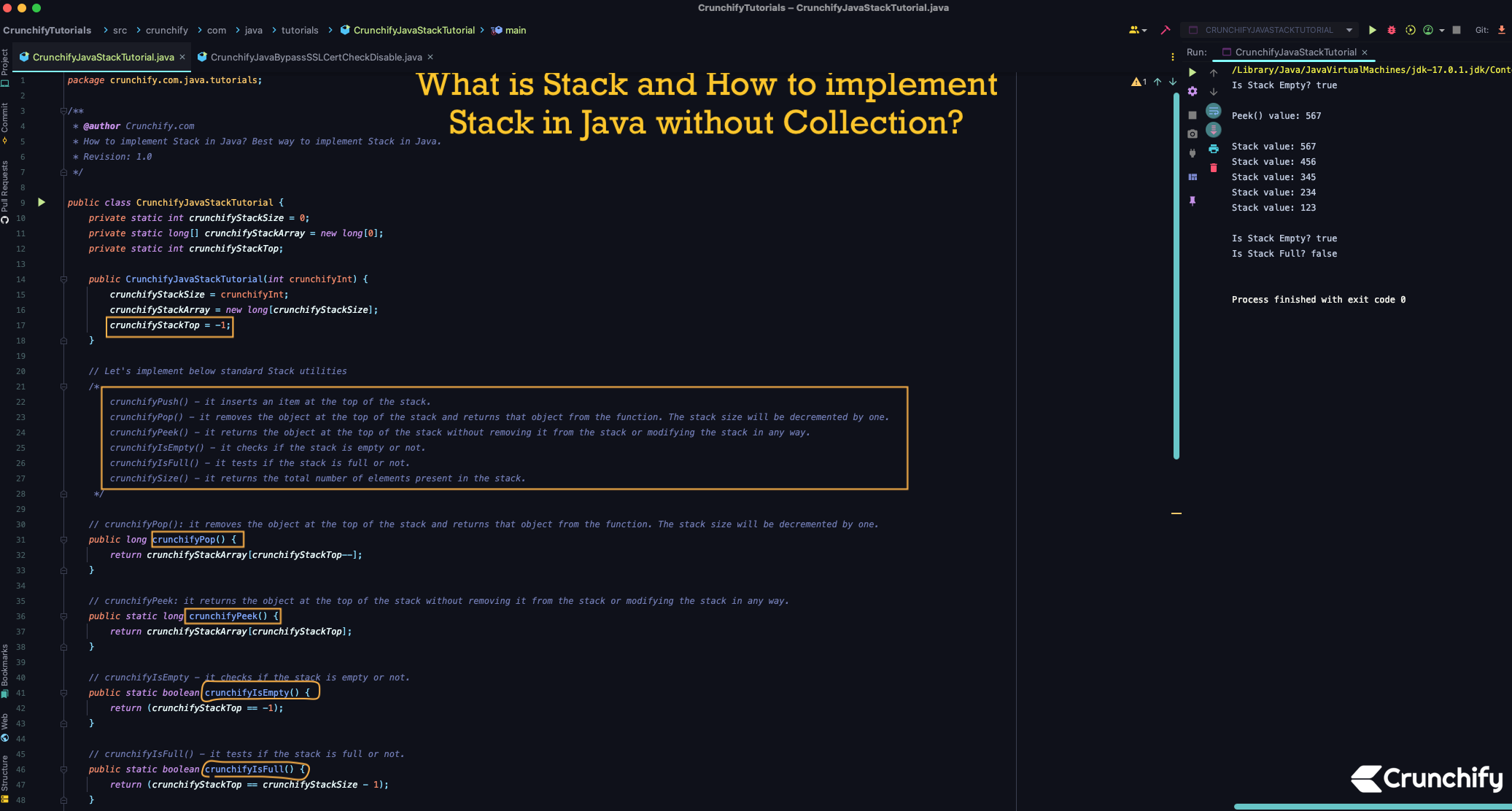
Was ist Stack in Java?
Haben Sie schon von LIFO gehört? Last-In, First-Out-Konzept? Nun, Stack ist eine LIFO-Implementierung der linearen Datenstruktur. Das heißt, Objekte können nur von einem Ende ODER also nur von oben eingefügt oder entfernt werden.
Hier ist unsere eigene Implementierung von Stack in Java
Wir werden die folgenden Funktionen für Java Stack erstellen. Bitte beachten Sie hier: Wir verwenden keine eingebaute Java-Collection-Klasse für die Stack-Implementierung.
Wir werden Java Collection for Stack im nächsten Tutorial verwenden. Es ist jetzt Artikellink.
- crunchifyPush() – fügt ein Element oben in den Stapel ein.
- crunchifyPop() – es entfernt das Objekt an der Spitze des Stapels und gibt dieses Objekt von der Funktion zurück. Die Stapelgröße wird um eins verringert.
- crunchifyPeek () – es gibt das Objekt an der Spitze des Stapels zurück, ohne es aus dem Stapel zu entfernen oder den Stapel in irgendeiner Weise zu ändern.
- crunchifyIsEmpty() – prüft, ob der Stack leer ist oder nicht.
- crunchifyIsFull() – testet ob der Stack voll ist oder nicht.
- crunchifySize() – gibt die Gesamtzahl der im Stack vorhandenen Elemente zurück.
Lass uns anfangen:
- Erstellen Sie die Klasse CrunchifyJavaStackTutorial.java
- Kopieren Sie den folgenden Code in Ihre Eclipse- oder IntelliJ-IDEE.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 |
package crunchify . com . java . tutorials ; /** * @author Crunchify.com * How to implement Stack in Java? Best way to implement Stack in Java. * Revision: 1.0 */ public class CrunchifyJavaStackTutorial { private static int crunchifyStackSize = 0 ; private static long [ ] crunchifyStackArray = new long [ 0 ] ; private static int crunchifyStackTop ; public CrunchifyJavaStackTutorial ( int crunchifyInt ) { crunchifyStackSize = crunchifyInt ; crunchifyStackArray = new long [ crunchifyStackSize ] ; crunchifyStackTop = - 1 ; } // Let's implement below standard Stack utilities /* crunchifyPush() - it inserts an item at the top of the stack. crunchifyPop() - it removes the object at the top of the stack and returns that object from the function. The stack size will be decremented by one. crunchifyPeek() - it returns the object at the top of the stack without removing it from the stack or modifying the stack in any way. crunchifyIsEmpty() - it checks if the stack is empty or not. crunchifyIsFull() - it tests if the stack is full or not. crunchifySize() - it returns the total number of elements present in the stack. */ // crunchifyPop(): it removes the object at the top of the stack and returns that object from the function. The stack size will be decremented by one. public long crunchifyPop ( ) { return crunchifyStackArray [ crunchifyStackTop -- ] ; } // crunchifyPeek: it returns the object at the top of the stack without removing it from the stack or modifying the stack in any way. public static long crunchifyPeek ( ) { return crunchifyStackArray [ crunchifyStackTop ] ; } // crunchifyIsEmpty - it checks if the stack is empty or not. public static boolean crunchifyIsEmpty ( ) { return ( crunchifyStackTop == - 1 ) ; } // crunchifyIsFull() - it tests if the stack is full or not. public static boolean crunchifyIsFull ( ) { return ( crunchifyStackTop == crunchifyStackSize - 1 ) ; } // crunchifyPush() - it inserts an item at the top of the stack. public void crunchifyPush ( long j ) { crunchifyStackArray [ ++ crunchifyStackTop ] = j ; } // crunchifySize() - it returns the total number of elements present in the stack. public static int crunchifySize ( ) { return crunchifyStackTop + 1 ; } public static void main ( String [ ] crunchifyArgs ) { CrunchifyJavaStackTutorial crunchifyStack = new CrunchifyJavaStackTutorial ( 5 ) ; crunchifyPrint ( "Is Stack Empty? " + crunchifyIsEmpty ( ) + "\n" ) ; crunchifyStack . crunchifyPush ( 123 ) ; crunchifyStack . crunchifyPush ( 234 ) ; crunchifyStack . crunchifyPush ( 345 ) ; crunchifyStack . crunchifyPush ( 456 ) ; crunchifyStack . crunchifyPush ( 567 ) ; crunchifyPrint ( "Peek() value: " + crunchifyPeek ( ) + "\n" ) ; while ( ! crunchifyIsEmpty ( ) ) { long crunchifyValue = crunchifyStack . crunchifyPop ( ) ; crunchifyPrint ( "Stack value: " + crunchifyValue ) ; } crunchifyPrint ( "" ) ; crunchifyPrint ( "Is Stack Empty? " + crunchifyIsEmpty ( ) ) ; crunchifyPrint ( "Is Stack Full? " + crunchifyIsFull ( ) + "\n" ) ; } // Simple Crunchify Print Utility private static void crunchifyPrint ( Object crunchifyValue ) { System . out . println ( crunchifyValue ) ; } } |
Java-Programm ausführen:
Führen Sie einfach das obige Programm als Java-Anwendung aus und Sie sollten das Ergebnis wie unten sehen.

1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
Is Stack Empty ? true Peek ( ) value : 567 Stack value : 567 Stack value : 456 Stack value : 345 Stack value : 234 Stack value : 123 Is Stack Empty ? true Is Stack Full ? false Process finished with exit code 0 |
Lassen Sie mich wissen, wenn Sie Fragen haben oder eine Ausnahme beim Ausführen des obigen Java-Programms erhalten, und ich bin mehr als glücklich, dies mit Ihnen zu debuggen.